Create Composite Structure Diagram Using Open API
A composite structure diagram is a member of the UML family, which shows the internal structure of a structured classifier or collaboration. This article will show you how to create a composite structure diagram using Open API.
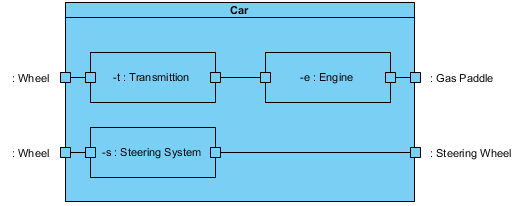
The composite structure diagram will be created by the plugin
Create Blank Diagram
First we create a blank diagram using DiagramManager.createDiagram().
//Create blank diagram DiagramManager diagramManager = ApplicationManager.instance().getDiagramManager(); ICompositeStructureDiagramUIModel composite = (ICompositeStructureDiagramUIModel) diagramManager.createDiagram(IDiagramTypeConstants.DIAGRAM_TYPE_COMPOSITE_STRUCTURE_DIAGRAM); composite.setName("Sample Composite Structure Diagram");
Create Model
In a composite structure diagram, we will need to create a model from IModelElementFactory, then set the diagram a sub diagram of the model using IModel.assSubDiagram().
//Create model IModel model = IModelElementFactory.instance().createModel(); model.setName("Sample Composite Structure Diagram - Car"); //Add the diagram to the model model.addSubDiagram(composite);
Create Class
Once the model is created, we can create a class from IModelElementFactory.
//Create Class IClass classCar = IModelElementFactory.instance().createClass(); classCar.setName("Car"); classCar.setVisibility(IClass.VISIBILITY_PUBLIC); //make the class a child the model model.addChild(classCar); //Create shape on diagram IBaseStructuredClassUIModel shapeClassCar = (IBaseStructuredClassUIModel) diagramManager.createDiagramElement(composite, classCar); shapeClassCar.setBounds(100, 50, 350, 200); shapeClassCar.resetCaption();
Create Parts (Attributes)
We can create the parts once the class is created. For every part, we will first need to create a class (no shape), then the attribute. Please keep in mind that the model and shape classes for a part are IAttribute and IPartUIModel respectively.
//Create Part Transmission //Create the class of the part IClass classTransmission = IModelElementFactory.instance().createClass(); classTransmission.setName("Transmission"); classTransmission.setVisibility(IClass.VISIBILITY_PUBLIC); //make the part's class a child of the model model.addChild(classTransmission); //Create the Attribute of the part IAttribute partTransmission = IModelElementFactory.instance().createAttribute(); partTransmission.setName("t"); partTransmission.setVisibility(IAttribute.VISIBILITY_PRIVATE); partTransmission.setType(classTransmission); partTransmission.setScope(IAttribute.SCOPE_INSTANCE); partTransmission.setAggregation(IAttribute.AGGREGATION_COMPOSITED); //Add the attribute to the corresponding class classCar.addAttribute(partTransmission); //Create the shape on diagram IPartUIModel shapePartTransmission = (IPartUIModel) diagramManager.createDiagramElement(composite, partTransmission); shapePartTransmission.setBounds(125, 100, 125, 50); shapePartTransmission.resetCaption(); //Make the shape as a child of the shape Car shapeClassCar.addChild(shapePartTransmission);
Create Ports for the Part
Once a part is created, we must create the associated ports immediately using IAttribute.createPort().
//Create Ports for Transmission //Create the port from the part IPort portTransmissionLeft = partTransmission.createPort(); portTransmissionLeft.setVisibility(IPort.VISIBILITY_PUBLIC); portTransmissionLeft.setService(true); //Create shape on diagram IPortUIModel shapePortTransmissionLeft= (IPortUIModel) diagramManager.createDiagramElement(composite, portTransmissionLeft); shapePortTransmissionLeft.setBounds(120, 120, 10, 10); //Make the port as a child of the part shapePartTransmission.addChild(shapePortTransmissionLeft);
Create Ports for the Class
When all parts and their ports are all created, we can then create the remaining ports. As these posts are not associated to any parts, a class will need to be created for each port.
//Create Ports for Car and their Classes //Create Class for the Port IClass classWheel = IModelElementFactory.instance().createClass(); classWheel.setName("Wheel"); classWheel.setVisibility(IClass.VISIBILITY_PUBLIC); //Make the class a child of the model model.addChild(classWheel); //Create Port IPort portWheel1 = classCar.createPort(); portWheel1.setVisibility(IPort.VISIBILITY_PUBLIC); portWheel1.setType(classWheel); portWheel1.setService(true); //Create shape on diagram IPortUIModel shapePortWheel1 = (IPortUIModel) diagramManager.createDiagramElement(composite, portWheel1); shapePortWheel1.setBounds(95, 120, 10, 10); shapePortWheel1.resetCaption(); //make the caption displayed on the left hand side (west -> left) shapePortWheel1.getCaptionUIModel().setSide(ICaptionUIModel.SIDE_WEST); //Make the port shape as a child of the car's shape shapeClassCar.addChild(shapePortWheel1);
Create Associations
We can now create associations once all classes, parts and ports are created.
//Create Association connecting Ports together IAssociation wheelToTransmition = IModelElementFactory.instance().createAssociation(); //This association is connecting from the port wheel1... wheelToTransmition.setFrom(portWheel1); // the port on the left hand side of attribute transmission wheelToTransmition.setTo(portTransmissionLeft); //Create connector on diagram diagramManager.createConnector(composite, wheelToTransmition, shapePortWheel1, shapePortTransmissionLeft, new Point[] {new Point(105,125), new Point(120,125)});
Sample Plugin
The sample plugin demonstrate how to create composite structure diagram using Open API. After you deploy the plugin into Visual Paradigm you can then click the plugin button in the application toolbar to trigger it.
Download Sample Diagram
You can click here to download the diagram.
Related Know-how |
Related Link |
Leave a Reply
Want to join the discussion?Feel free to contribute!