Create a Breakdown Structure Diagram Using Open API
Breakdown structures are commonly used in project management when there are needs in visualizing parent-to-child relationship. This article will show you how to create a breakdown structure diagram using Open API.
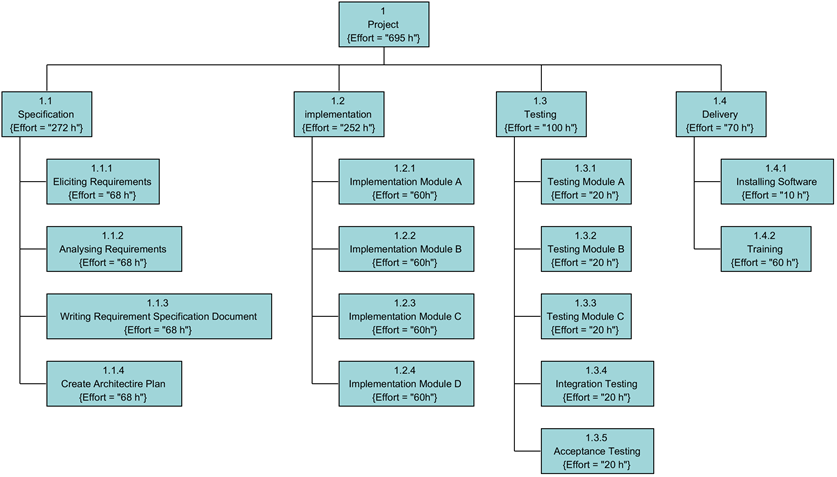
The breakdown structure diagram will be created by the plugin.
Create Blank Breakdown Structure Diagram
A blank breakdown structure diagram can be first created using DiagramManager.createDiagram.
//Create blank diagram DiagramManager diagramManager = ApplicationManager.instance().getDiagramManager(); IBreakdownStructureUIModel breakdown = (IBreakdownStructureUIModel) diagramManager.createDiagram(IDiagramTypeConstants.DIAGRAM_TYPE_BREAKDOWN_STRUCTURE); breakdown.setName("Sample Breakdown Structure Diagram"); breakdown.setShowTaggedValues(true);
Create Title
Once the blank diagram is created, we can then create the title, which is an element, from IModelElementFactory.
//Create the Title IBSElement titleProject = IModelElementFactory.instance().createBSElement(); titleProject.setName("Project"); titleProject.setCode(1); //Create the element shape on the diagram IBSElementUIModel shapeTitleProject = (IBSElementUIModel) diagramManager.createDiagramElement(breakdown, titleProject); shapeTitleProject.setBounds(425, 25, 100, 50); shapeTitleProject.resetCaption();
Create Elements and Sub-Ordinates
Except the title, every element in a breakdown structure diagram is considered as a sub-ordinates (children) of another element. Thus, a element can be created by the method IBSElement.createBSElement().
//Create Specification (One of the sub-ordinates of the title) //This is a sub-ordinate of the element Project IBSElement specification = titleProject.createBSElement(); specification.setName("Specification"); specification.setCode(1); //Create the element shape on the diagram IBSElementUIModel shapeSpecification = (IBSElementUIModel) diagramManager.createDiagramElement(breakdown, specification); shapeSpecification.setBounds(50, 125, 100, 50); shapeSpecification.resetCaption();
Create Tagged values for elements
To assign a tagged value to an element, you will first need a tagged value container created from IModelElementFactory. Then you can create a tagged value using ITaggedValueContainer.createTaggedValue(). Afterwards, the element will be assigned the container using method IBSElement.setTaggedvalues().
//Create tagged value container ITaggedValueContainer tvContainerProject = IModelElementFactory.instance().createTaggedValueContainer(); //Create tagged value ITaggedValue tvProject = tvContainerProject.createTaggedValue(); tvProject.setName("Effort"); tvProject.setType(ITaggedValue.TYPE_TEXT); tvProject.setValue("695 h"); //Assign the element a tagged value container titleProject.setTaggedValues(tvContainerProject);
For convenient, you can also create a private method under the java class, which can be used for multiple times. Here is an example of using a private method:
//Another way to create tagged values using private method //newTaggedVal() is a private method that returns a tagged value container specification.setTaggedValues(newTaggedVal("Effort", ITaggedValue.TYPE_TEXT, "272 h")); elicRequirements.setTaggedValues(newTaggedVal("Effort", ITaggedValue.TYPE_TEXT, "68 h")); anaRequirements.setTaggedValues(newTaggedVal("Effort", ITaggedValue.TYPE_TEXT, "68 h")); wriReqSpecDoc.setTaggedValues(newTaggedVal("Effort", ITaggedValue.TYPE_TEXT, "68 h")); creArcPlan.setTaggedValues(newTaggedVal("Effort", ITaggedValue.TYPE_TEXT, "68 h"));
And there is an example the private method:
private ITaggedValueContainer newTaggedVal(String name, int type, String value) { //Create a tagged value container ITaggedValueContainer container = IModelElementFactory.instance().createTaggedValueContainer(); //Create a tagged value in the container ITaggedValue taggedVal = container.createTaggedValue(); taggedVal.setName(name); taggedVal.setType(type); taggedVal.setValue(value); return container; }
Create Connectors
When the elements and sub-ordinates are created, we are ready to create some connectors using DiagramManager.createConnector().
//Create connectors from the title diagramManager.createConnector(breakdown, IBreakdownStructureUIModel.SHAPETYPE_BS_CONNECTOR, shapeTitleProject, shapeSpecification, null); diagramManager.createConnector(breakdown, IBreakdownStructureUIModel.SHAPETYPE_BS_CONNECTOR, shapeTitleProject, shapeImplementation, null); diagramManager.createConnector(breakdown, IBreakdownStructureUIModel.SHAPETYPE_BS_CONNECTOR, shapeTitleProject, shapeTesting, null); diagramManager.createConnector(breakdown, IBreakdownStructureUIModel.SHAPETYPE_BS_CONNECTOR, shapeTitleProject, shapeDelivery, null);
Show up Diagram
Finally, show up the diagram.
//Show up diagram diagramManager.openDiagram(breakdown);
Sample Plugin
The sample plugin demonstrate how to create breakdown structure diagram using Open API. After you deploy the plugin into Visual Paradigm you can then click the plugin button in the application toolbar to trigger it.
Download Sample Plugin
You can download the sample plugin here.
Related Know-how |
Related Link |
Leave a Reply
Want to join the discussion?Feel free to contribute!