Create a Sequence Diagram Using Open API
Sequence diagram is a member of the UML diagram family, which is mainly used to show the interactions between object through time using a various lifelines. In this article, you will be shown how to create a sequence diagram from scratch using Open API.
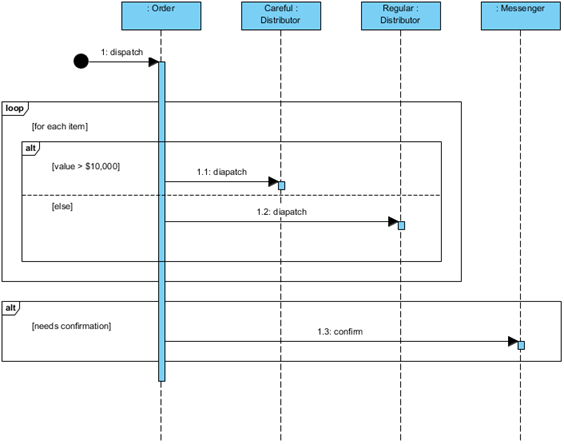
The sequence diagram will be created by the plugin.
Create Blank Diagram
First create a blank diagram using DiagramManager.createDiagram().
//Create blank diagram DiagramManager diagramManager = ApplicationManager.instance().getDiagramManager(); IInteractionDiagramUIModel sequence = (IInteractionDiagramUIModel) diagramManager.createDiagram(IDiagramTypeConstants.DIAGRAM_TYPE_INTERACTION_DIAGRAM); sequence.setName("Sample Sequence Diagram"); // Retrieve root frame of the interaction IFrame rootFrame = sequence.getRootFrame(true);
Create Model
Once the diagram is created, create a model. Then, make the diagram a sub diagram of the model using IModel.addSubDiagram().
//Craete Model IModel model = IModelElementFactory.instance().createModel(); model.setName("diagram model"); model.addSubDiagram(sequence);
Create Classes
Before creating any lifeline, you will need to create the corresponding class for every lifeline from IModelElementFactory.
//Create Classes IClass classOrder = IModelElementFactory.instance().createClass(); classOrder.setName("Order"); classOrder.setVisibility(IClass.VISIBILITY_PUBLIC); model.addChild(classOrder);
Create Lifelines
After all classes are created, you can now use IModelElementfactory to create some timelines. Do not forget to add the a class as a lifeline’s base classifier using IInteractionLifeLine.setBaseClassifier().
//Create Lifelines IInteractionLifeLine lifelineOrder = IModelElementFactory.instance().createInteractionLifeLine(); // Add lifeline to root frame rootFrame.addChild(lifelineOrder); lifelineOrder.setName(""); //declare which class does this lifeline associates to lifelineOrder.setBaseClassifier(classOrder); //Create shape on diagram IInteractionLifeLineUIModel shapeOrder = (IInteractionLifeLineUIModel) diagramManager.createDiagramElement(sequence, lifelineOrder); shapeOrder.setBounds(200, 25, 100, 550); shapeOrder.resetCaption();
Create Activation
Once the lifelines are created, you can create activation from IModelElementFactory. Remember to use IInteractionLifeLine.addActivation() to make the activation a child of a lifeline.
//Create activation IActivation activationOrder = IModelElementFactory.instance().createActivation(); //make this activation a part of the life line Order lifelineOrder.addActivation(activationOrder); //Create shape on diagram IActivationUIModel shapeActivationOrder = (IActivationUIModel) diagramManager.createDiagramElement(sequence, activationOrder); shapeActivationOrder.setBounds(246, 100, IActivationUIModel.BODY_WIDTH, 400);
Create Operands
We can create some operands from IModelElementFactory. We will also have to create a constraint for each operands. Once the constraint is created, it will then be assigned to a operands using IInteractionOperand.setGuard().
//Create operands IInteractionOperand eachItem = IModelElementFactory.instance().createInteractionOperand(); eachItem.setName("each Item"); //Create constraint IInteractionConstraint constraintEachItem = IModelElementFactory.instance().createInteractionConstraint(); constraintEachItem.setName("for each item"); constraintEachItem.setConstraint("for each item"); //add the constraint into the operand eachItem.setGuard(constraintEachItem); //Create shape on diagram IInteractionOperandUIModel shapeEachItem = (IInteractionOperandUIModel) diagramManager.createDiagramElement(sequence, eachItem);
Create Combined Fragments
We can now create some combined fragments once the operands are created. You will need to state the covering lifelines and the used operands with ICombinedFragment.addCoveredLifeLine() and ICombinedFragment.addOperand() respectively.
//Create Combine fragments ICombinedFragment fragmentEachItem = IModelElementFactory.instance().createCombinedFragment(); fragmentEachItem.setName("Loop for each item"); rootFrame.addChild(fragmentEachItem); //This is a loop fragmentEachItem.setInteractionOperator(ICombinedFragment.INTERACTION_OPERATOR_LOOP); //Give the fragment an operand fragmentEachItem.addOperand(eachItem); //this fragment will cover three life lines fragmentEachItem.addCoveredLifeLine(lifelineOrder); fragmentEachItem.addCoveredLifeLine(lifelineCareful); fragmentEachItem.addCoveredLifeLine(lifelineRegular); //Create shape on diagram ICombinedFragmentUIModel shapeFragmentEachItem = (ICombinedFragmentUIModel) diagramManager.createDiagramElement(sequence, fragmentEachItem); shapeFragmentEachItem.setBounds(50, 150, 575, 225); //add the operand shape as child of the fragment shapeFragmentEachItem.addChild(shapeEachItem); shapeFragmentEachItem.resetCaption();
Create Messages and Found/Lost Messages
You can create a message from IModelElementFactory. If it is a found/lost message, you will need to create a lost/found message end shape using IInteractionDiagramUIModel.createDiagramElement().
This is how you create a message:
//Create messages IMessage message1_1 = IModelElementFactory.instance().createMessage(); message1_1.setName("diapatch"); message1_1.setSequenceNumber("1.1"); //This message will connect from the activation on the Order time line... message1_1.setFromActivation(activationOrder); //... to the Careful time line. message1_1.setToActivation(activationCareful); //make the message a child of the value > operand such that the message will move with the operand's combined fragment value.addMessage(message1_1); //Create shape on diagram IMessageUIModel shapeMessage1_1 = (IMessageUIModel) diagramManager.createConnector(sequence, message1_1, shapeOrder, shapeCareful, new Point[] {new Point(254,250), new Point(396,250)}); shapeMessage1_1.resetCaption();
And for creating a found message:
//Create found message //Create found/lost shape ILostFoundMessageEndShapeUIModel found = (ILostFoundMessageEndShapeUIModel) sequence.createDiagramElement(IInteractionDiagramUIModel.SHAPETYPE_LOST_FOUND_MESSAGE_END); found.setBounds(140, 90, 20, 20); //Create message IMessage message1 = IModelElementFactory.instance().createMessage(); message1.setName("dispatch"); message1.setSequenceNumber("1"); //This message will connect to the activation on the Order life line message1.setToActivation(activationOrder); //Create shape on diagram IMessageUIModel shapeMessage1 = (IMessageUIModel) diagramManager.createConnector(sequence, message1, found, shapeOrder, new Point[] {new Point(160,100), new Point(246,100)}); shapeMessage1.resetCaption();
Show Diagram
Finally, show up the diagram
//show diagram diagramManager.openDiagram(sequence);
Sample Plugin
The sample plugin demonstrate how to create sequence diagram using Open API. After you deploy the plugin into Visual Paradigm you can then click the plugin button in the application toolbar to trigger it.
Download Sample Plugin
You can click this link to download the sample plugin.
Related Know-how |
Related Link |
Leave a Reply
Want to join the discussion?Feel free to contribute!