Create Data Flow Diagram Using Open API
A Data Flow Diagram displays the flow of data of a system, using rectangles and arrows. This article will demonstrate how to create a data flow diagram using Open API.
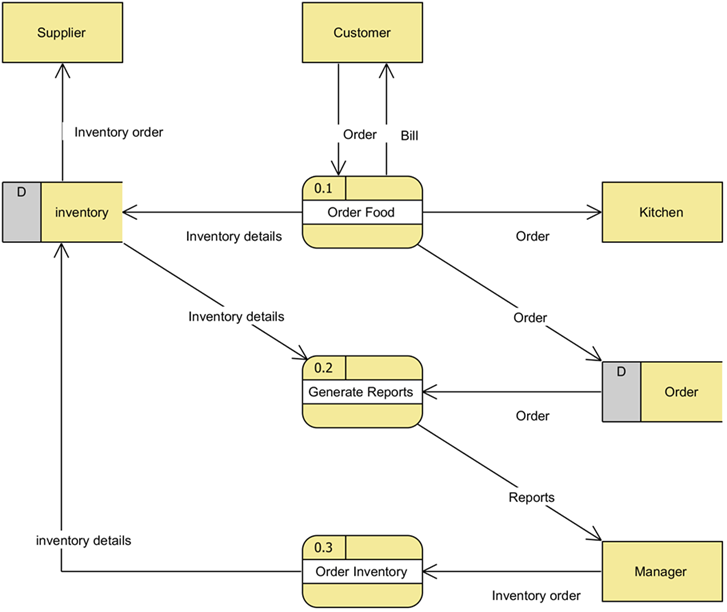
The diagram will be created by the plugin
Create Blank Data Flow Diagram
First we use DiagramManager.createDiagram to create a blank diagram.
//Create Blank DFD DiagramManager diagrammanager = ApplicationManager.instance().getDiagramManager(); IDiagramUIModel dfd = (IDiagramUIModel) diagrammanager.createDiagram(IDiagramTypeConstants.DIAGRAM_TYPE_DATA_FLOW_DIAGRAM); dfd.setName("Simple Data Flow Diagram");
Create Processes, Entities and Data Stores
A process, entity and data store can all be created once the diagram is created. We can use IModelElementFactory to create processes, external entities and data stores.
This is how you create a process:
//Create Order Food Process IDFProcess orderFood = IModelElementFactory.instance().createDFProcess(); orderFood.setName("Order Food"); orderFood.setDfId("0.1"); //Create the process shape on diagram IDFProcessUIModel shapeOrderFood = (IDFProcessUIModel) diagrammanager.createDiagramElement(dfd, orderFood); shapeOrderFood.setBounds(450, 395, 100, 60);
This is how you create an external entity:
//Create External Entity IDFExternalEntity customer = IModelElementFactory.instance().createDFExternalEntity(); customer.setName("Customer"); //Create the external entity on diagram IDFExternalEntityUIModel shapeCustomer = (IDFExternalEntityUIModel) diagrammanager.createDiagramElement(dfd, customer); shapeCustomer.setBounds(450, 200, 100, 50);
This is how you create a data store:
//Create Data Store IDFDataStore inventory = IModelElementFactory.instance().createDFDataStore(); inventory.setName("inventory"); //Create data store on diagram IDFDataStoreUIModel shapeInventory = (IDFDataStoreUIModel) diagrammanager.createDiagramElement(dfd, inventory); shapeInventory.setBounds(200, 400, 100, 50);
You can try repeating these steps to create more processes, external entities and data stores.
Create Data Flows
The way to create a data flow is similar to a process, entity and data store. However, rather than using the DiagramManager.createDiagramElement() method, DiagramManager.createConnector() is being used instead.
In order the name to the flow be seen, the method IDFDataFlowUIModel.resetCaption() has to be used.
First set up the model for the data flow:
//Create Data Flow IDFDataFlow customerOrderFood = IModelElementFactory.instance().createDFDataFlow(); customerOrderFood.setName("Order"); //The data flow is connecting from the customer external entity... customerOrderFood.setFrom(customer); //...to the order food process customerOrderFood.setTo(orderFood);
Then create the shape on the diagram, do not forget resetting the caption:
//Create the data flow on diagram //If new Point[] is empty, the data flow will be created automatically IDFDataFlowUIModel shapeCustomerOrderFood = (IDFDataFlowUIModel) diagrammanager.createConnector(dfd, customerOrderFood, shapeCustomer, shapeOrderFood, new Point[] {new Point(480,250), new Point(480,395)}); shapeCustomerOrderFood.resetCaption();
Now try to create other data flows by repeating these steps.
Show Up the Diagram
Finally, show up the diagram.
// Show up the diagram diagrammanager.openDiagram(dfd);
Sample Plugin
The sample plugin demonstrate how to create data flow diagram using Open API. After you deploy the plugin into Visual Paradigm you can then click the plugin button in the application toolbar to trigger it.
Download the Plugin
You can click this link to download the sample plugin.
Related Know-how |
Related Link |
Leave a Reply
Want to join the discussion?Feel free to contribute!