Create Entity-Relationship Diagram Using Open API
Entity-relationship modelling (ER model) becomes handy when building a data base. An ER diagram is being used to indicate relations between different entities in a data base. This article will show you how to create an Entity-Relationship Diagram using Open API.
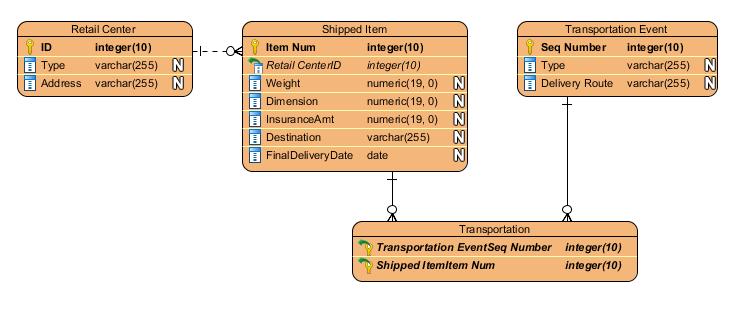
The ERD going to create by the plugin
Create Blank Entity-Relationship Diagram
A blank diagram can be created by using DiagramManager.createDiagram.
//Create blank ER Diagram DiagramManager diagrammanager = ApplicationManager.instance().getDiagramManager(); IDiagramUIModel erd = (IDiagramUIModel) diagrammanager.createDiagram(IDiagramTypeConstants.DIAGRAM_TYPE_ER_DIAGRAM); erd.setName("Simple ER Diagram");
Create Tables (Entities) and Their Columns (Attributes)
We are ready to create tables and columns once the diagram is made. IModelElementFactory becomes handy when creating the Retail Center table.
//Create table for retail centers IDBTable tableRetailCenter = IModelElementFactory.instance().createDBTable(); tableRetailCenter.setName("Retail Center"); //Create the table shape on diagram IDBTableUIModel shapeRetailCenter = (IDBTableUIModel) diagrammanager.createDiagramElement(erd, tableRetailCenter); shapeRetailCenter.setBounds(50,50,175,75); //Call to re-calculate caption position when render the diagram shapeRetailCenter.resetCaption();
One the table is created, we can use the IDBTable.createDBColumn() method to add columns into the table. The type, length and scale of a column’s are set up using the IDBColumn.setType() method.
//Create the ID column under Retail Center table IDBColumn retailCenterID = tableRetailCenter.createDBColumn(); retailCenterID.setName("ID"); //Make it a primary key/ a member of a composite key retailCenterID.setPrimaryKey(true); //This attribute cannot be empty in any record retailCenterID.setNullable(false); //The attribute is an Integer with maximum 10 digits with no scale retailCenterID.setType("integer", 10, 0);
If a column contains a primary key, or a member of a composite key, the method IDBColumn.setPrimary() has to be used.
//Make it a primary key/ a member of a composite key retailCenterID.setPrimaryKey(true);
Create Foreign Keys, Relationships and Connectors
Every foreign key has to be created by using IModelElementFactory. The corresponding relationship will also be defined during the process.
//Create a foreign key IDBForeignKey shippedItemRetailCenterID = IModelElementFactory.instance().createDBForeignKey(); //The foreign key is connecting from the retail Center table... shippedItemRetailCenterID.setFrom(tableRetailCenter); //to the Shipped Item table shippedItemRetailCenterID.setTo(tableShippedItem); //Create a constraint IDBForeignKeyConstraint RetailCenterIdConstraint = IModelElementFactory.instance().createDBForeignKeyConstraint(); //The new foreign key will be referencing the ID column in the Retail Center table RetailCenterIdConstraint.setRefColumn(retailCenterID); RetailCenterIdConstraint.setForeignKey(shippedItemRetailCenterID);
A relationship can be visualised by a connector, using the DiagramManager.createConnector() method.
//Create the connector diagrammanager.createConnector(erd, shippedItemRetailCenterID, shapeRetailCenter, shapeShippedItem, new Point[] {new Point (225,80), new Point (275,80)});
Create Columns which are Both Primary and Foreign Key
If a column’s attribute is both a primary and foreign key, do not create a column under the table. Instead, first create a foreign key, then use the method IDBForeignKey.setIdentifying(). Do not forget to visualize the connection.
//Create a foreign key as usual IDBForeignKey transportationTransportationSeqNum = IModelElementFactory.instance().createDBForeignKey(); transportationTransportationSeqNum.setFrom(TableTransportationEvent); transportationTransportationSeqNum.setTo(tableItemTransportation); IDBForeignKeyConstraint seqNumConstraint = IModelElementFactory.instance().createDBForeignKeyConstraint(); seqNumConstraint.setRefColumn(transportationEventSeqNumber); seqNumConstraint.setForeignKey(transportationTransportationSeqNum); //Make the key both Primary and Foreign transportationTransportationSeqNum.setIdentifying(true); //connector diagrammanager.createConnector(erd, transportationShippedItemNum, shapeShippedItem, shapeItemTransportation, new Point[] {new Point (425,200), new Point (425,250)});
Show Up the Diagram
Finally we can show up the diagram.
// Show up the diagram diagrammanager.openDiagram(erd);
Sample Plugin
The sample plugin demonstrate how to create ER diagram using Open API. After you deploy the plugin into Visual Paradigm you can then click the plugin button in the application toolbar to trigger it.
Download the Plugin
You can click this link to download the sample plugin.
Related Know-how |
Related Link |
Leave a Reply
Want to join the discussion?Feel free to contribute!