Create ArchiMate diagram Using Open API
ArchiMate is a modelling technique for describing enterprise architectures. This article will show you how to create an ArchiMate diagram using Open API.
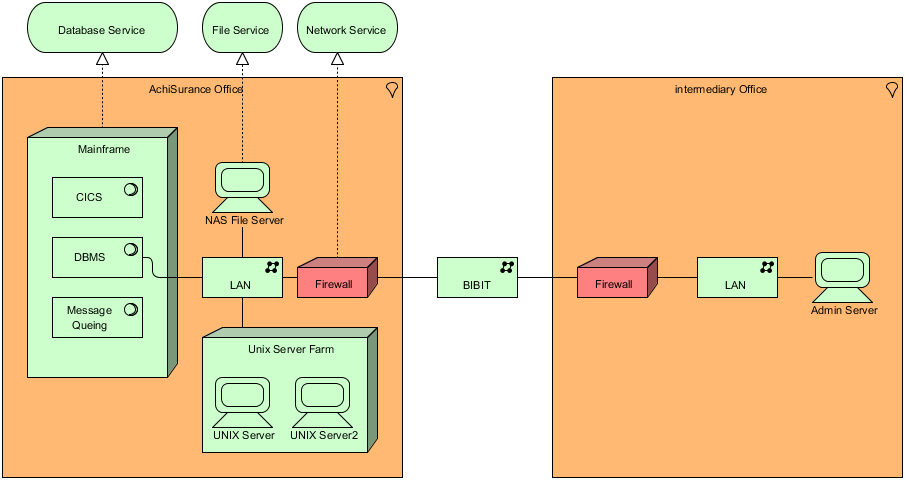
The ArchiMate diagram will be created by the plugin
Create Blank Diagram
First we use DiagramManager.createDiagram to create a blank diagram.
DiagramManager diagramManager = ApplicationManager.instance().getDiagramManager(); IArchiMateDiagramUIModel archiMate = (IArchiMateDiagramUIModel) diagramManager.createDiagram(IDiagramTypeConstants.DIAGRAM_TYPE_ARCHI_MATE_DIAGRAM); archiMate.setName("Sample ArchiMate Diagram");
Create Locations
Once the diagram is created, we can create locations from IModelElementFactory.
//Create Locations IArchiMateLocation archiSuranceOffice = IModelElementFactory.instance().createArchiMateLocation(); archiSuranceOffice.setName("AchiSurance Office"); //Create shape for location on diagram IArchiMateLocationUIModel shapeArchiSuranceOffice = (IArchiMateLocationUIModel) diagramManager.createDiagramElement(archiMate, archiSuranceOffice); shapeArchiSuranceOffice.setBounds(50, 100, 400, 400); shapeArchiSuranceOffice.resetCaption();
Create Nodes
We are free to create some nodes from IModelElementFactory when the locations are created.
//Create Nodes IArchiMateNode mainframe = IModelElementFactory.instance().createArchiMateNode(); mainframe.setName("Mainframe"); // Add Mainframe to become child of ArchiSurance Office archiSuranceOffice.addChild(mainframe); //Create shape on diagram IArchiMateNodeUIModel shapeMainframe = (IArchiMateNodeUIModel) diagramManager.createDiagramElement(archiMate, mainframe); shapeMainframe.setBounds(75, 150, 150, 250); //show the node as a symbol instead of a box shapeMainframe.setShowOption(IArchiMateNodeUIModel.SHOW_OPTION_AS_SYMBOL); shapeArchiSuranceOffice.addChild(shapeMainframe); shapeMainframe.resetCaption();
Create System software and devices
We can now use IArchiMateNode.createArchiMateSystemSoftware() and IArchiMateNode.createArchiMateDevice() to create some system software and devices.
To create a system software:
//Create System software IArchiMateSystemSoftware cics = mainframe.createArchiMateSystemSoftware(); cics.setName("CICS"); //Create shape on diagram IArchiMateSystemSoftwareUIModel shapeCics = (IArchiMateSystemSoftwareUIModel) diagramManager.createDiagramElement(archiMate, cics); shapeCics.setBounds(100, 200, 90, 40); //Show the shape as a box instead of a symbol shapeCics.setShowOption(IArchiMateSystemSoftwareUIModel.SHOW_OPTION_AS_BOX); shapeMainframe.addChild(shapeCics); shapeCics.resetCaption();
To create a device:
//Create devices IArchiMateDevice unixServer1 = unixServerFarm.createArchiMateDevice(); unixServer1.setName("UNIX Server"); unixServerFarm.addArchiMateDevice(unixServer1); //Create shape on diagram IArchiMateDeviceUIModel shapeUnixServer1 = (IArchiMateDeviceUIModel) diagramManager.createDiagramElement(archiMate, unixServer1); shapeUnixServer1.setBounds(260, 400, 60, 50); shapeUnixServer1.setShowOption(IArchiMateDeviceUIModel.SHOW_OPTION_AS_SYMBOL); shapeUnixServerFarm.addChild(shapeUnixServer1); shapeUnixServer1.resetCaption(); //Set the caption to be shown below the shape shapeUnixServer1.getCaptionUIModel().setSide(ICaptionUIModel.SIDE_SOUTH);
Create network boxes
No we can use IModelElementFactory to create some network boxes.
//Create network box IArchiMateNetworkBox lan = IModelElementFactory.instance().createArchiMateNetworkBox(); lan.setName("LAN"); //Create a shape on diagram IArchiMateNetworkBoxUIModel shapeLanMaster = (IArchiMateNetworkBoxUIModel) diagramManager.createDiagramElement(archiMate, lan); shapeLanMaster.setBounds(250, 280, 80, 40); shapeArchiSuranceOffice.addChild(shapeLanMaster); shapeLanMaster.resetCaption(); //Create another shape on the diagram IArchiMateNetworkBoxUIModel shapeLanAbstract = (IArchiMateNetworkBoxUIModel) diagramManager.createDiagramElement(archiMate, lan); shapeLanAbstract.setBounds(745, 280, 80, 40); shapeIntermediaryOffice.addChild(shapeLanAbstract); shapeLanAbstract.resetCaption(); //Set the first shape as Master view shapeLanMaster.toBeMasterView();
Create Services
Now, use IModelElementFactory to create some services.
//Create Service IArchiMateInfrastructureService databaseService = IModelElementFactory.instance().createArchiMateInfrastructureService(); databaseService.setName("Database Service"); //Create shape on diagram IArchiMateInfrastructureServiceUIModel shapeDatabaseService = (IArchiMateInfrastructureServiceUIModel) diagramManager.createDiagramElement(archiMate, databaseService); shapeDatabaseService.setBounds(75, 25, 150, 50); shapeDatabaseService.resetCaption();
Create associations and realizations
When all elements are created, we are off to create associations and realizations from IModelElementFactory.
To create an associations:
//Create Service IArchiMateInfrastructureService databaseService = IModelElementFactory.instance().createArchiMateInfrastructureService(); databaseService.setName("Database Service"); //Create shape on diagram IArchiMateInfrastructureServiceUIModel shapeDatabaseService = (IArchiMateInfrastructureServiceUIModel) diagramManager.createDiagramElement(archiMate, databaseService); shapeDatabaseService.setBounds(75, 25, 150, 50); shapeDatabaseService.resetCaption();
To create a realizations:
// Create realizations IArchiMateRealization mainframeToDatabaseService = IModelElementFactory.instance().createArchiMateRealization(); //The realization starts from mainframe... mainframeToDatabaseService.setFrom(mainframe); //... to databaseService mainframeToDatabaseService.setTo(databaseService); //Draw connection on diagram diagramManager.createConnector(archiMate, mainframeToDatabaseService,shapeMainframe, shapeDatabaseService, new Point[] {new Point(150, 150), new Point(150, 75) });
Show Diagram
Finally, open the diagram.
/ Show up diagram diagramManager.openDiagram(archiMate);
Sample Plugin
The sample plugin demonstrate how to create business process diagram using Open API. After you deploy the plugin into Visual Paradigm you can then click the plugin button in the application toolbar to trigger it.
Download Sample Plugin
You can click this link to download the sample plugin.
Related Know-how |
Related Link |
Leave a Reply
Want to join the discussion?Feel free to contribute!